Actor#
Actor is a component of Pipeline
, that contains the Script
and handles it.
It is responsible for processing user input and determining the appropriate response based
on the current state of the conversation and the script.
The actor receives requests in the form of a Context
class, which contains
information about the user’s input, the current state of the conversation, and other relevant data.
The actor uses the dialog graph, represented by the Script
class,
to determine the appropriate response. The script contains the structure of the conversation,
including the different nodes and transitions.
It defines the possible paths that the conversation can take, and the conditions that must be met
for a transition to occur. The actor uses this information to navigate the graph
and determine the next step in the conversation.
Overall, the actor acts as a bridge between the user’s input and the dialog graph, making sure that the conversation follows the expected flow and providing a personalized experience to the user.
Below you can see a diagram of user request processing with Actor.
Both request and response are saved to Context
.
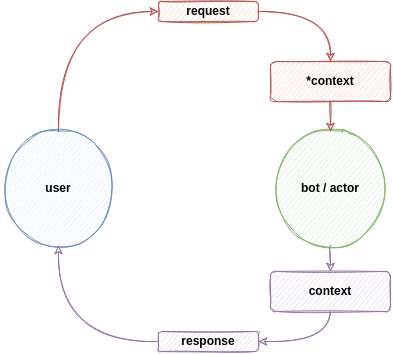
- error_handler(error_msgs, msg, exception=None, logging_flag=True)[source]#
This function handles errors during
Script
validation.- Parameters:
error_msgs (
list
) – List that contains error messages.error_handler()
adds every next error message to that list.msg (
str
) – Error message which is to be added into error_msgs.exception (
Optional
[Exception
]) – Invoked exception. If it has been set, it is used to obtain logging traceback. Defaults to None.logging_flag (
bool
) – The flag which defines whether logging is necessary. Defaults to True.
- class Actor(script, start_label, fallback_label=None, label_priority=1.0, condition_handler=None, handlers=None)[source]#
Bases:
object
The class which is used to process
Context
according to theScript
.- Parameters:
script (
Union
[Script
,dict
]) – The dialog scenario: a graph described by theKeywords
. While the graph is being initialized, it is validated and then used for the dialog.start_label (
Tuple
[str
,str
]) – The start node ofScript
. The execution begins with it.fallback_label (
Optional
[Tuple
[str
,str
]]) – The label ofScript
. Dialog comes into that label if all other transitions failed, or there was an error while executing the scenario. Defaults to None.label_priority (
float
) – Default priority value for alllabels
where there is no priority. Defaults to 1.0.condition_handler (
Optional
[Callable
]) – Handler that processes a call of condition functions. Defaults to None.handlers (
Optional
[Dict
[ActorStage
,List
[Callable
]]]) –This variable is responsible for the usage of external handlers on the certain stages of work of
Actor
.key (
ActorStage
) - Stage in which the handler is called.value (List[Callable]) - The list of called handlers for each stage. Defaults to an empty dict.
- _overwrite_node(current_node, flow_label, only_current_node_transitions=False)[source]#
- Return type:
- async run_response(response, ctx, pipeline)[source]#
Executes the normalized response as an asynchronous function. See the details in the
normalize_response()
function of normalization.py.- Return type:
- async _run_processing_parallel(processing, ctx, pipeline)[source]#
Execute the processing functions for a particular node simultaneously, independent of the order.
Picked depending on the value of the
Pipeline
’s parallelize_processing flag.- Return type:
None
- async _run_processing_sequential(processing, ctx, pipeline)[source]#
Execute the processing functions for a particular node in-order.
Picked depending on the value of the
Pipeline
’s parallelize_processing flag.- Return type:
None
- async _run_pre_transitions_processing(ctx, pipeline)[source]#
Run PRE_TRANSITIONS_PROCESSING functions for a particular node. Pre-transition processing functions can modify the context state before the direction of the next transition is determined depending on that state.
The execution order depends on the value of the
Pipeline
’s parallelize_processing flag.- Return type:
None
- async _run_pre_response_processing(ctx, pipeline)[source]#
Run PRE_RESPONSE_PROCESSING functions for a particular node. Pre-response processing functions can modify the response before it is returned to the user.
The execution order depends on the value of the
Pipeline
’s parallelize_processing flag.- Return type:
None